Also see Pieter Blignaut's notes on
OOP Programming in Delphi
An object is regarded as an instance of a
class. Therefore a class can be viewed as a framework for an object.
Classes are defined like this:
type
TClass_Name = class
fFieldName1 : data_type;
fFieldName2 : data_type;
procedure Procedure_Name;
function Function_Name : data_type;
end;
var
Object_Name : TClass_Name
EXAMPLE
This example makes use of a class called TMovies that includes the fields
fName, fGenre and fDuration (in minutes). It also includes the function
getDuration that calculates the duration hours and minutes.
Typically when using Classes these steps are followed:
- Add the class definition
- Create reference to description of object
- Create an object
- Create functions/procedures
- Set values in object
- Call function/procedure and display
- Free space in memory
Follow these steps when creating your own unit:
- Create a new unit
- Put structure of class in Interface section of new unit
- Put code for method(s) in the Implementation section
- Add new unit to main program?s uses clause
- Save and Execute
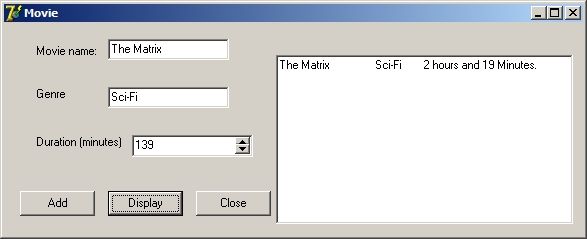
MAIN PROGRAM: Unit1.pas
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls,
Forms,
Dialogs, StdCtrls, ComCtrls, Spin, MoviesClass;
//Add new unit to main program?s uses
clause
type
TfrmFilms = class(TForm)
Edit1: TEdit;
Label1: TLabel;
Label2: TLabel;
Label3: TLabel;
Edit2: TEdit;
SpinEdit1: TSpinEdit;
Button1: TButton;
RichEdit1: TRichEdit;
Button2: TButton;
Button3: TButton;
procedure Button1Click(Sender: TObject);
procedure FormCreate(Sender: TObject);
procedure Button3Click(Sender: TObject);
procedure Button2Click(Sender: TObject);
private
{ Private declarations }
public
{ Public declarations }
end;
var
frmFilms: TfrmFilms;
Movies : TMovies;
implementation
{$R *.dfm}
{ TMovie }
procedure TfrmFilms.FormCreate(Sender: TObject);
begin
Movies := TMovies.Create;
end;
procedure TfrmFilms.Button1Click(Sender: TObject);
begin
Movies.fName := Edit1.Text;
Movies.fGenre := Edit2.Text;
Movies.fDuration := SpinEdit1.Value;
end;
procedure TfrmFilms.Button2Click(Sender: TObject);
var
sOutput : String;
begin
sOutput := Movies.getDuration;
RichEdit1.Lines.Add(sOutput);
end;
procedure TfrmFilms.Button3Click(Sender: TObject);
begin
Movies.Free;
//Destructor: frees memory
Application.Terminate;
end;
end.
CLASS IN OWN UNIT:
MovieClass.pas
unit MoviesClass;
//Create a new unit
interface
uses SysUtils;
//This is added to be able to use IntToStr
type
//Put structure of class in Interface
section of new unit
TMovies = class
fName : String[25];
fGenre : String[10];
fDuration : Integer;
function getDuration: String;
constructor Create;
end;
implementation //Put
code for method(s) in the Implementation section
constructor TMovies.Create;
//Reserve memory for object
begin
inherited Create;
fName := '';
fGenre := '';
fDuration := 0;
end;
function TMovies.getDuration: String;
var
iHour, iMin : Integer;
sDuration : String;
begin
iHour := fDuration DIV 60;
iMin := fDuration - (iHour * 60);
sDuration := IntToStr(iHour) + ' hours and ' + IntToStr(iMin) + '
Minutes.';
Result := fName + #9 + fGenre + #9 + sDuration;
end;
end. |
Also see the Example
Class question as well as question 2 in the
Grade 12 exemplar.
Sources consulted
- Object Oriented Programming in Delphi - A Guide for Beginners
http://www.webtechcorp.co.uk/web-developer-training-delphi-article-oop.htm
- Object Oriented Programming in Delphi. Pieter Blignaut (Jan. 2008)
- Enjoy Delphi. Part 2. A. Bezuidenhout, K. Gibson, C. Noom?, U.
Wassermann, M. Zeeman (S. Jacobs, ed.) (2005) - p. 207-252
- Creative Programming in Delphi. Part 2. M. Havenga & C. Moraal. (2007) -
p. 135-156
- Introducing Delphi Programming: Theory through practice. J. Barrow, L.
Miller, K. Malan & H. Gelderblom. (2005) ? p. 486-520 |